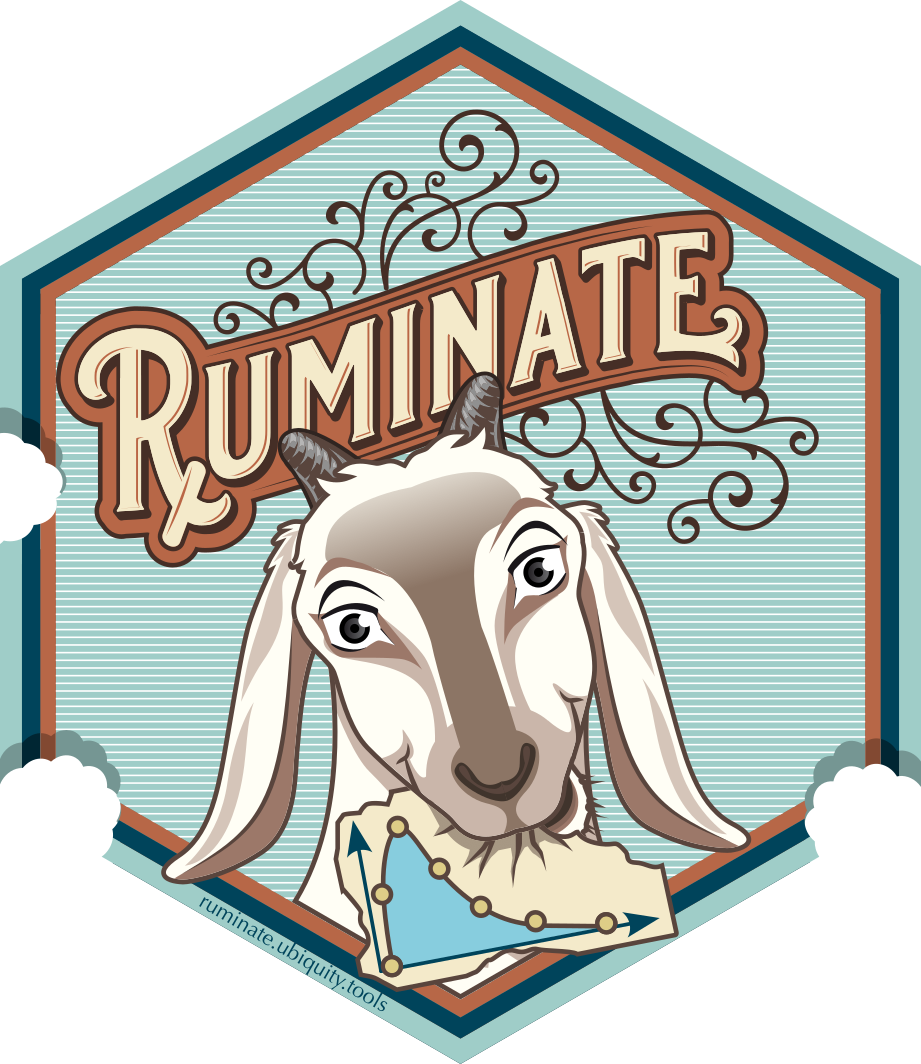
Creates Tables of Individual Observations from PKNCA Result
Source:R/NCA_Server.R
mk_table_ind_obs.Rd
Takes the output of PKNCA and creates a tabular view of the individual observation data. This can be spread out of over several tables (pages) if necessary.
Usage
mk_table_ind_obs(
nca_res,
obnd = NULL,
not_sampled = "NS",
blq = "BLQ",
digits = 3,
text_format = "text",
max_height = 7,
max_width = 6.5,
max_row = NULL,
max_col = 9,
notes_detect = NULL,
rows_by = "time"
)
Arguments
- nca_res
Output of PKNCA.
- obnd
onbrand reporting object.
- not_sampled
Character string to use for missing data when pivoting.
- blq
Character string to use to indicate data below the level of quantification (value of 0 in the dataset).
- digits
Number of significant figures to report (set to
NULL
to disable rounding)- text_format
Either
"md"
for markdown or"text"
(default) for plain text.- max_height
Maximum height of the final table in inches (A value of
NULL
will use 100 inches).- max_width
Maximum width of the final table in inches (A value of
NULL
will use 100 inches).- max_row
Maximum number of rows to have on a page. Spillover will hang over the side of the page..
- max_col
Maximum number of columns to have on a page. Spillover will be wrapped to multiple pages.
- notes_detect
Vector of strings to detect in output tables (example
c("NC", "BLQ")
).- rows_by
Can be either "time" or "id". If it is "time", there will be a column for time and separate column for each subject ID. If rows_by is set to "id" there will be a column for ID and a column for each individual time.
Value
List containing the following elements
isgood: Boolean indicating the exit status of the function.
one_table: Dataframe of the entire table with the first lines containing the header.
one_body: Dataframe of the entire table (data only).
one_header: Dataframe of the entire header (row and body, no data).
tables: Named list of tables. Each list element is of the output
msgs: Vector of text messages describing any errors that were found. format from
build_span
.
Examples
# We need a state variable to be define
sess_res = NCA_test_mksession()
#> → ASM: including file
#> → ASM: source: file.path(system.file(package="onbrand"), "templates", "report.docx")
#> → ASM: dest: file.path("config","report.docx")
#> → ASM: including file
#> → ASM: source: file.path(system.file(package="onbrand"), "templates", "report.pptx")
#> → ASM: dest: file.path("config","report.pptx")
#> → ASM: including file
#> → ASM: source: file.path(system.file(package="onbrand"), "templates", "report.yaml")
#> → ASM: dest: file.path("config","report.yaml")
#> → ASM: State initialized
#> → ASM: module isgood: TRUE
#> → UD: including file
#> → UD: source: file.path(system.file(package="onbrand"), "templates", "report.docx")
#> → UD: dest: file.path("config","report.docx")
#> → UD: including file
#> → UD: source: file.path(system.file(package="onbrand"), "templates", "report.pptx")
#> → UD: dest: file.path("config","report.pptx")
#> → UD: including file
#> → UD: source: file.path(system.file(package="onbrand"), "templates", "report.yaml")
#> → UD: dest: file.path("config","report.yaml")
#> → UD: State initialized
#> → UD: module checksum updated:897d952fecbc804999396a96f9df4b20
#> → UD: module isgood: TRUE
#> → DW: including file
#> → DW: source: file.path(system.file(package="onbrand"), "templates", "report.docx")
#> → DW: dest: file.path("config","report.docx")
#> → DW: including file
#> → DW: source: file.path(system.file(package="onbrand"), "templates", "report.pptx")
#> → DW: dest: file.path("config","report.pptx")
#> → DW: including file
#> → DW: source: file.path(system.file(package="onbrand"), "templates", "report.yaml")
#> → DW: dest: file.path("config","report.yaml")
#> → DW: State initialized
#> → DW: module checksum updated:5b0f0b05ee3ac7336a74c564bb6efdad
#> → DW: loading data view idx: 1
#> → DW: setting name: Observations
#> → DW: module checksum updated:1ac3e0afcc601f848943f92b854b3830
#> → DW: -> filter
#> → DW: module checksum updated:67aff6e926eba73b3ecb361d26624844
#> → DW: -> filter
#> → DW: module checksum updated:0234f6d458ef7487a3b2e991b2d0957b
#> → DW: -> mutate
#> → DW: module checksum updated:37d0958f042076b974fdd3f894157822
#> → DW: loading data view idx: 2
#> → DW: setting name: PK 3mg SD IV
#> → DW: module checksum updated:4e7ca05728d66df3adfcf87387f9543a
#> → DW: -> filter
#> → DW: module checksum updated:2d95bc56262f46b7f135dd33fc4722b3
#> → DW: -> filter
#> → DW: module checksum updated:b8a30837145926d2d9cc6f788c10b7ed
#> → DW: -> filter
#> → DW: module checksum updated:24b554ee8736ebc84979c896ac2e93c3
#> → DW: -> filter
#> → DW: module checksum updated:daad88263faf7cbf90871ed00a0dd275
#> → DW: loading data view idx: 3
#> → DW: setting name: PK 3mg SD IV (NCA)
#> → DW: module checksum updated:a7b3ea35cdba272682716aa4619d3983
#> → DW: -> filter
#> → DW: module checksum updated:e8d2f77c0bd731995d845ee8be623e22
#> → DW: -> filter
#> → DW: module checksum updated:24cc7b591ca756a0abf560fb5295e04d
#> → DW: -> filter
#> → DW: module checksum updated:f79ef26bdb4dac420c24650b9e96f721
#> → DW: loading data view idx: 4
#> → DW: setting name: PKPD 3mg SD IV (NCA)
#> → DW: module checksum updated:c737e8b3a28ba600a83ddf58a969c90a
#> → DW: -> filter
#> → DW: module checksum updated:4c0d054f8ec47ae97fd1c650d8ddad55
#> → DW: -> filter
#> → DW: module checksum updated:1e3d65ec1bd6ee5850d67bd2250e3223
#> → DW: -> filter
#> → DW: module checksum updated:1e3d65ec1bd6ee5850d67bd2250e3223
#> → DW: module isgood: TRUE
#> → NCA: including file
#> → NCA: source: file.path(system.file(package="onbrand"), "templates", "report.docx")
#> → NCA: dest: file.path("config","report.docx")
#> → NCA: including file
#> → NCA: source: file.path(system.file(package="onbrand"), "templates", "report.pptx")
#> → NCA: dest: file.path("config","report.pptx")
#> → NCA: including file
#> → NCA: source: file.path(system.file(package="onbrand"), "templates", "report.yaml")
#> → NCA: dest: file.path("config","report.yaml")
#> ✖ NCA: Parameter specified in YAML is not a valid PKNCA parameter: sparse_se
#> ✖ NCA: Parameter specified in YAML is not a valid PKNCA parameter: sparse_df
#> → NCA: including file
#> → NCA: source: file.path(system.file(package="onbrand"), "templates", "report.docx")
#> → NCA: dest: file.path("config","report.docx")
#> → NCA: including file
#> → NCA: source: file.path(system.file(package="onbrand"), "templates", "report.pptx")
#> → NCA: dest: file.path("config","report.pptx")
#> → NCA: including file
#> → NCA: source: file.path(system.file(package="onbrand"), "templates", "report.yaml")
#> → NCA: dest: file.path("config","report.yaml")
#> → NCA: State initialized
#> → NCA: State initialized
#> → NCA: loading element idx: 1
#> → NCA: -> setting name: PK Example
#> → NCA: -> notes found and set
#> → NCA: -> setting data source: DW_myDS_3
#> → NCA: NCA_add_int: append
#> → NCA: NCA_add_int: append
#> → NCA: added element idx: 1
#> → NCA: loading element idx: 2
#> → NCA: -> setting name: PK/PD Example
#> → NCA: -> notes found and set
#> → NCA: -> setting data source: DW_myDS_4
#> → NCA: NCA_add_int: append
#> → NCA: NCA_add_int: append
#> → NCA: added element idx: 2
#> → NCA: module isgood: TRUE
state = sess_res$state
# Pulls out the active analysis
current_ana = NCA_fetch_current_ana(state)
# This is the raw PKNCA output
pknca_res = NCA_fetch_ana_pknca(state, current_ana)
# Building the figure
mk_res = mk_table_ind_obs(nca_res = pknca_res)
mk_res$tables[["Table 1"]]$ft
Time
Analyte
Concentration (ng/mL) by ID
(day)
1
2
3
4
5
6
0
BM_ng_ml
1250
1030
831
1170
1140
868
1
BM_ng_ml
1650
1160
932
1340
1500
988
2
BM_ng_ml
1950
1240
1010
1450
1740
1080
21
BM_ng_ml
2280
1500
1130
1690
2190
1310
28
BM_ng_ml
2070
1440
1070
1610
2060
1250
3
BM_ng_ml
2170
1300
1070
1530
1900
1140
35
BM_ng_ml
1890
1380
1010
1530
1930
1190
4
BM_ng_ml
2330
1340
1110
1590
2020
1200
42
BM_ng_ml
1760
1320
972
1460
1810
1130
7
BM_ng_ml
2580
1430
1180
1690
2200
1290
0
C_ng_ml
BLQ
BLQ
BLQ
BLQ
BLQ
BLQ
1
C_ng_ml
690
750
670
638
855
611
2
C_ng_ml
588
577
576
517
668
521
21
C_ng_ml
123
274
139
173
228
194
28
C_ng_ml
95.5
220
104
135
188
155
3
C_ng_ml
506
508
501
438
549
456
35
C_ng_ml
75.8
177
77.8
105
156
125
4
C_ng_ml
438
476
441
384
472
409
42
C_ng_ml
60.6
142
58.6
82.2
128
100
7
C_ng_ml
303
425
320
300
360
325